How Developers Can Improve Their Debugging Techniques By Gustavo Woltmann
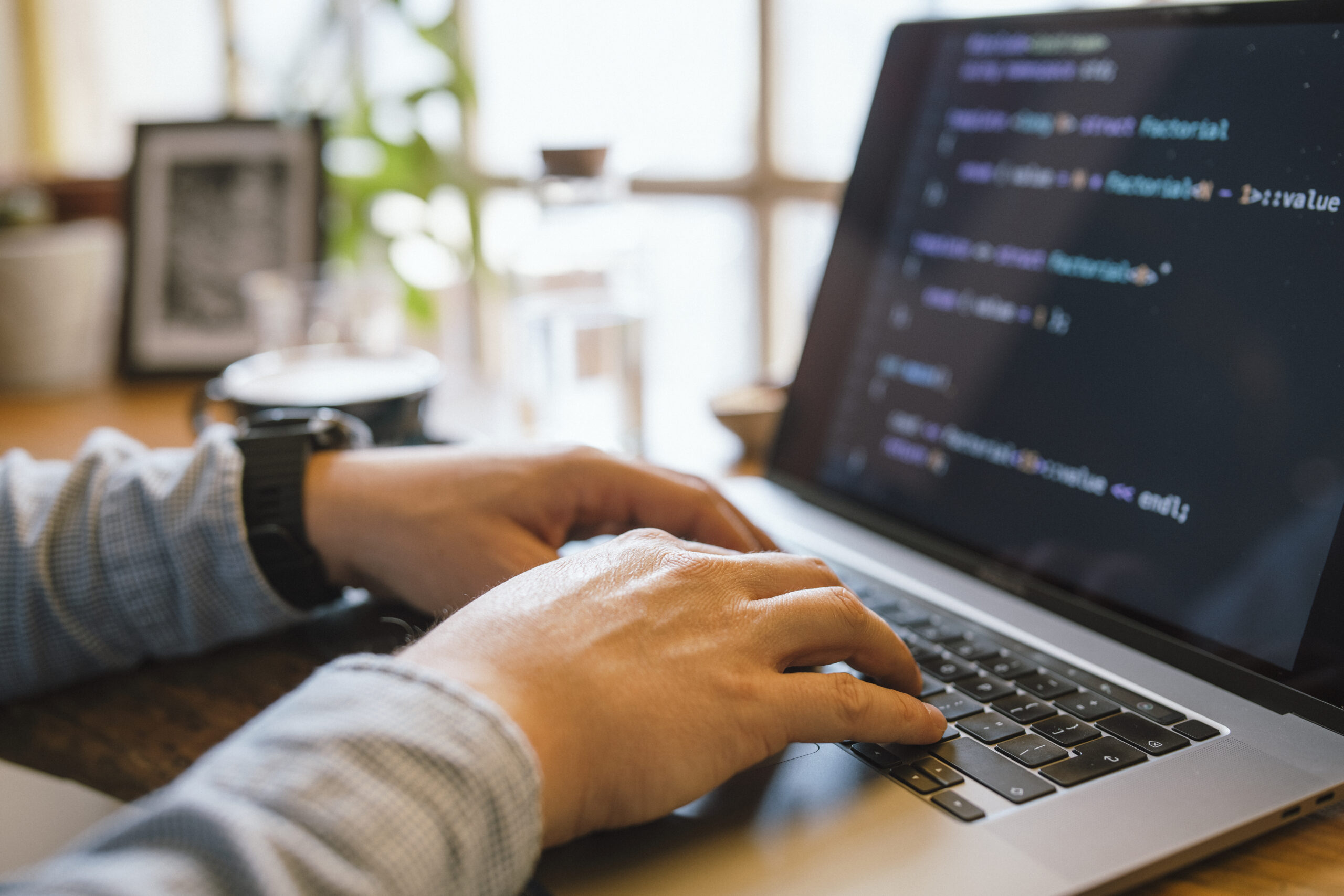
Debugging is Among the most essential — but generally missed — skills inside a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and learning to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can conserve hours of aggravation and significantly boost your productivity. Listed here are a number of methods to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest ways builders can elevate their debugging capabilities is by mastering the instruments they use everyday. When producing code is just one Section of growth, being aware of the best way to interact with it correctly through execution is equally important. Fashionable growth environments arrive Geared up with highly effective debugging capabilities — but many builders only scratch the floor of what these resources can perform.
Consider, for example, an Built-in Growth Surroundings (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications assist you to established breakpoints, inspect the value of variables at runtime, move by way of code line by line, and also modify code on the fly. When applied appropriately, they Permit you to observe accurately how your code behaves through execution, that is a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-finish builders. They permit you to inspect the DOM, observe network requests, watch actual-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can convert irritating UI difficulties into manageable duties.
For backend or system-degree builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over managing procedures and memory management. Understanding these instruments can have a steeper Studying curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into at ease with version Manage techniques like Git to be familiar with code history, locate the exact minute bugs ended up released, and isolate problematic changes.
In the end, mastering your resources signifies going beyond default settings and shortcuts — it’s about building an intimate familiarity with your progress ecosystem so that when problems arise, you’re not misplaced at nighttime. The higher you recognize your instruments, the greater time it is possible to commit fixing the particular difficulty as an alternative to fumbling by way of the method.
Reproduce the trouble
The most essential — and often ignored — steps in effective debugging is reproducing the condition. Right before leaping in the code or producing guesses, developers need to produce a regular natural environment or circumstance in which the bug reliably appears. Without the need of reproducibility, repairing a bug gets a video game of probability, typically leading to wasted time and fragile code changes.
The initial step in reproducing a challenge is gathering as much context as feasible. Check with queries like: What actions triggered The problem? Which surroundings was it in — improvement, staging, or output? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it results in being to isolate the exact disorders beneath which the bug occurs.
As soon as you’ve collected enough facts, make an effort to recreate the condition in your local ecosystem. This might necessarily mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, take into account crafting automated assessments that replicate the sting circumstances or point out transitions involved. These exams not simply help expose the challenge but will also avoid regressions Sooner or later.
Sometimes, the issue can be environment-certain — it would materialize only on particular working devices, browsers, or under certain configurations. Working with tools like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms may be instrumental in replicating these bugs.
Reproducing the problem isn’t only a action — it’s a state of mind. It needs endurance, observation, in addition to a methodical method. But as you can continually recreate the bug, you are previously midway to correcting it. Which has a reproducible state of affairs, You should use your debugging resources a lot more efficiently, examination likely fixes safely and securely, and talk a lot more Obviously along with your crew or consumers. It turns an abstract complaint into a concrete challenge — and that’s where by builders thrive.
Read and Understand the Mistake Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes Erroneous. In lieu of observing them as aggravating interruptions, developers should master to deal with error messages as direct communications within the process. They generally let you know precisely what happened, wherever it took place, and occasionally even why it happened — if you know the way to interpret them.
Commence by studying the information meticulously and in comprehensive. Quite a few developers, specially when beneath time stress, look at the 1st line and right away start building assumptions. But deeper during the error stack or logs may lie the real root trigger. Don’t just duplicate and paste error messages into search engines — examine and realize them first.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line selection? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Mastering to recognize these can dramatically increase your debugging procedure.
Some problems are imprecise or generic, and in People cases, it’s vital to look at the context wherein the error occurred. Check out similar log entries, input values, and recent alterations from the codebase.
Don’t overlook compiler or linter warnings either. These typically precede much larger issues and provide hints about prospective bugs.
In the long run, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, encouraging you pinpoint issues quicker, minimize debugging time, and become a far more successful and self-confident developer.
Use Logging Correctly
Logging is One of the more impressive tools in a developer’s debugging toolkit. When used successfully, it provides genuine-time insights into how an application behaves, assisting you realize what’s taking place under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts with knowing what to log and at what amount. Prevalent logging degrees involve DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for in-depth diagnostic information and facts through growth, Data for basic occasions (like successful get started-ups), Alert for prospective problems that don’t crack the applying, ERROR for actual complications, and Deadly once the system can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Too much logging can click here obscure vital messages and decelerate your method. Deal with critical activities, point out alterations, input/output values, and important selection points in the code.
Format your log messages clearly and continually. Contain context, such as timestamps, request IDs, and performance names, so it’s simpler to trace issues in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Particularly precious in production environments the place stepping through code isn’t attainable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, wise logging is about harmony and clarity. Which has a effectively-considered-out logging approach, you'll be able to lessen the time it takes to spot troubles, attain deeper visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized process—it is a method of investigation. To successfully recognize and deal with bugs, builders must method the method just like a detective fixing a thriller. This way of thinking helps break down complicated concerns into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering proof. Think about the symptoms of the issue: error messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, gather as much related details as you'll be able to without having jumping to conclusions. Use logs, test circumstances, and person reviews to piece collectively a clear picture of what’s happening.
Next, variety hypotheses. Talk to oneself: What could possibly be creating this behavior? Have any changes recently been built into the codebase? Has this challenge transpired prior to under identical situation? The purpose is always to narrow down possibilities and detect likely culprits.
Then, examination your theories systematically. Attempt to recreate the issue inside of a managed atmosphere. For those who suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, question your code queries and Allow the results guide you closer to the reality.
Shell out close notice to modest particulars. Bugs often cover within the the very least anticipated sites—just like a lacking semicolon, an off-by-one particular error, or possibly a race situation. Be extensive and affected person, resisting the urge to patch The difficulty with out absolutely knowing it. Temporary fixes may possibly hide the true trouble, only for it to resurface later on.
Lastly, retain notes on Everything you tried using and realized. Equally as detectives log their investigations, documenting your debugging process can conserve time for long run problems and enable Other people fully grasp your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, technique complications methodically, and turn out to be simpler at uncovering concealed issues in sophisticated units.
Create Assessments
Writing assessments is among the most effective solutions to improve your debugging capabilities and In general development efficiency. Exams not simply help catch bugs early but additionally serve as a safety Internet that provides you self confidence when earning changes for your codebase. A perfectly-tested software is much easier to debug because it enables you to pinpoint specifically in which and when a difficulty happens.
Begin with unit exams, which target particular person features or modules. These modest, isolated assessments can promptly expose no matter if a certain piece of logic is Functioning as anticipated. Whenever a check fails, you instantly know exactly where to look, significantly lessening some time expended debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear soon after Formerly becoming fixed.
Upcoming, combine integration exams and finish-to-close checks into your workflow. These support make certain that various aspects of your application work alongside one another efficiently. They’re especially useful for catching bugs that manifest in intricate methods with various parts or solutions interacting. If a little something breaks, your exams can tell you which Section of the pipeline failed and less than what problems.
Writing assessments also forces you to Assume critically about your code. To check a characteristic thoroughly, you may need to understand its inputs, envisioned outputs, and edge instances. This standard of knowing The natural way qualified prospects to raised code structure and much less bugs.
When debugging an issue, producing a failing test that reproduces the bug might be a robust first step. When the exam fails constantly, you may concentrate on repairing the bug and check out your check move when The difficulty is resolved. This strategy makes certain that the same bug doesn’t return Later on.
To put it briefly, creating assessments turns debugging from the frustrating guessing recreation right into a structured and predictable system—helping you catch a lot more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s uncomplicated to be immersed in the problem—looking at your display for hrs, striving Alternative after solution. But Just about the most underrated debugging equipment is simply stepping away. Taking breaks helps you reset your mind, decrease disappointment, and sometimes see The problem from the new point of view.
When you are far too near the code for far too very long, cognitive exhaustion sets in. You would possibly start out overlooking evident faults or misreading code you wrote just several hours previously. In this particular condition, your brain gets to be much less effective at problem-resolving. A brief stroll, a coffee break, or simply switching to another undertaking for 10–15 minutes can refresh your focus. Lots of builders report obtaining the basis of a problem when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support stop burnout, Particularly all through extended debugging periods. Sitting down before a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power in addition to a clearer frame of mind. You could suddenly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a good guideline is to established a timer—debug actively for forty five–60 minutes, then have a 5–ten minute split. Use that time to move all-around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specifically less than restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, having breaks just isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is part of fixing it.
Master From Each and every Bug
Just about every bug you encounter is more than simply A short lived setback—It is really an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you something beneficial should you make the effort to replicate and review what went wrong.
Begin by asking your self several essential inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved practices like device tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or knowledge and make it easier to build stronger coding habits going ahead.
Documenting bugs can even be an outstanding routine. Hold a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you figured out. After a while, you’ll start to see patterns—recurring challenges or prevalent faults—which you could proactively stay away from.
In group environments, sharing what you've acquired from the bug along with your peers is usually Specially effective. Whether or not it’s via a Slack concept, a short generate-up, or a quick understanding-sharing session, encouraging Some others stay away from the identical issue boosts staff effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your development journey. In spite of everything, a few of the most effective developers are certainly not the ones who produce ideal code, but people who consistently understand from their mistakes.
Ultimately, Each individual bug you resolve provides a brand new layer to the talent set. So upcoming time you squash a bug, have a second to mirror—you’ll occur away a smarter, far more able developer due to it.
Conclusion
Increasing your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It makes you a more productive, self-assured, and capable developer. The following time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.